Spring Boot – a project facilitating the development of applications in Java
The Spring Framework is the main development platform that makes Java development easier and more efficient. Spring itself is also described as a “lightweight infrastructure” due to its low dependence on APIs.
Read this article to find out:
- the nature of the Spring Boot project
- how applications are developed in Spring Boot
- the advantages of using this solution
The origins of Spring Boot
In pre-Spring Boot times, developers using the Spring framework had to ensure correct dependencies between the libraries they were adding. The incompatibility of libraries was a frequent problem during API configuration. An additional challenge was the need for an external server (e.g. Tomcat) to upload and validate the application. Configuration, uploading, running and testing the application was a laborious process. For this reason, a group of developers supporting the development of the Spring Framework decided to find a faster way to create new applications.
Using Spring Boot to build applications
In order to solve the previously defined problems, programmers created so-called “starters”. To put it simply, starters are a set of all required libraries and configurations. The set also has a built-in Tomcat application server. To start using Spring Boot, you just need to:
- add the appropriate dependencies to the pom.xml configuration file (if you use Maven)
- add a “parent” from which the application will inherit dependencies
Here’s an example of how little it takes to reap the benefits of all that Spring Boot has to offer:
<parent><span data-ccp-props="{}"> </span>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId><span data-ccp-props="{}"> </span>
<version>1.5.9.RELEASE</version><span data-ccp-props="{}"> </span>
</parent>
As you can see, Spring Boot will save you long hours you would otherwise spend dealing with the basic configuration.
The next thing you need to do is add starters to your dependencies; for example, the “spring-boot-starter-web”, which supplies libraries for web engines. Below is an example of an added dependency.
<dependencies><span data-ccp-props="{}">
</span> (...)<span data-ccp-props="{}">
</span> <dependency><span data-ccp-props="{}">
</span> <groupId>org.springframework.boot</groupId><span data-ccp-props="{}">
</span> <artifactId>spring-boot-starter-web</artifactId><span data-ccp-props="{}">
</span> </dependency><span data-ccp-props="{}">
</span> (...)<span data-ccp-props="{}">
</span></dependencies><span data-ccp-props="{}">
</span>
The last step in the process is to create a class, which will be responsible for running the application.
@SpringBootApplication<span data-ccp-props="{}"> </span>
<b>public</b> <b>class</b> ExampleApplication {<span data-ccp-props="{}"> </span>
<b> public</b> <b>static</b> <b>void</b> main(String[] args) {<span data-ccp-props="{}"> </span>
SpringApplication.<i>run</i>(ExampleApplication.<b>class</b>, args);<span data-ccp-props="{}"> </span>
}<span data-ccp-props="{}"> </span>
}<span data-ccp-props="{}"> </span><span data-ccp-props="{}">
</span>
Once the application is ready, you can launch it in two ways:
- through the development environment as a “Java application”
- from the command line (java-jar …)
To learn more, go to the official website of the Spring project.
Spring Boot adaptation in microservices
Noting the huge potential of their project, Spring Framework’s creators began to develop it very quickly. This process gave rise to an increasing number of Spring Boot starters. Why do applications based on Spring Boot favor the development of IT systems based on the architecture of microservices? Before answering this question, let me clarify what microservices are.
As the name suggests, microservices are tiny applications that perform a small portion of tasks relative to the whole IT system. They are designed to maximize their autonomy, with only weak links to other services. They should be run on separate hosts, as this ensures greater independence and helps avoid server overload and failures.
Microservices have a host of advantages, including:
- use of technology that accurately reflects business needs, e.g. you can use another programming language, a relational or non-relational NoSQL database, etc.
- services that will experience a heavy load from time to time (e.g. a reporting service) can be replicated, and traffic could be evenly distributed over individual nodes using Load Balancing tools, thus accelerating that respective part of the system.
- when adding new functionalities to the system, there is no need to restart the entire system hoping that no errors will occur. The new service can be implemented without any additional complications.
Back to the question: Why does Spring Boot favor the development of IT systems based on the architecture of microservices? With its simple configuration and quick set up, without unnecessary external application servers, Spring Boot can constantly provide customers with new functionalities. You are not tied to any single technology. You don’t need to stop the whole system, so its SLA-based availability is close to 100%.
Your first project in Spring Boot
Now it’s time to test your knowledge in practice. Below is the outline of a new project development – it’s worth taking a look at the tutorial prepared by Spring with a step by step description of how to create and run applications. The tutorial will show you three possible methods of how to create an application using Spring Boot:
- Via the website provided by Spring, where you can configure your project. Just fill in the required fields, select what you need to use, and click “Generate Project”. Done!
- Installing the IDE plugin, and developing a project there using the Spring Tool Suite.
- Generating the project by means of an appropriate tool, e.g. Maven.
Below is an example of a Spring Boot application created using the second method:
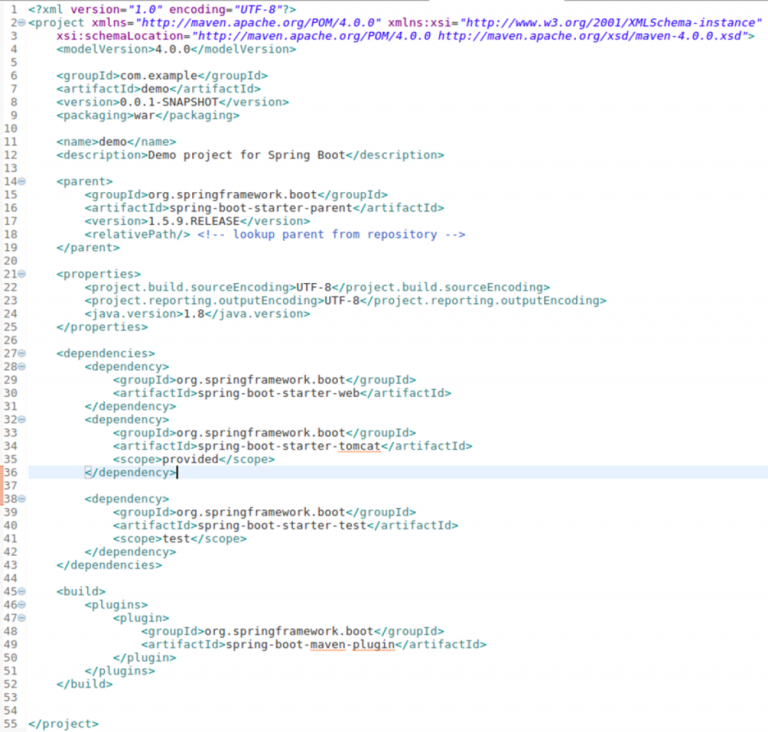
Next, go to the class which is responsible for launching your application.

Figure 2 shows a ready DemoApplication class with @SpringBootApplication and @RestController annotations. The first annotation replaces other annotations that are designed to run automatic configuration, scan components, and add additional configuration classes using one annotation only. To make the operation more convenient, a second note was added to this class. It informs the user that the class will also be a controller. In the DemoApplication class, you have the main method, which you can use to run the application. Lines 15–18 contain an example of a REST service implementation that returns a Message object in the default JSON format.
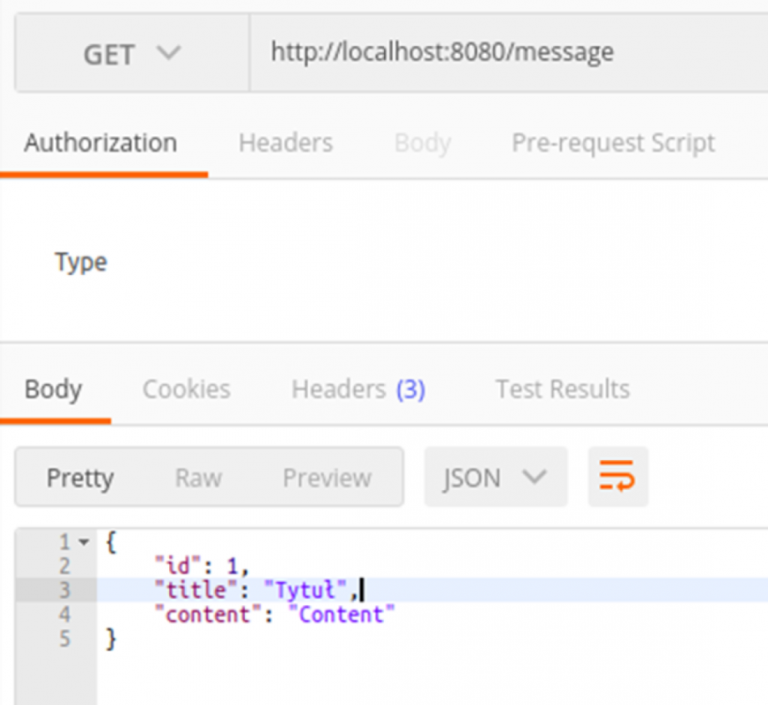
Figure 3 shows the result from the service that accurately reflects the prepared object.
Summary
The above features of a Spring Boot-based application are just a few of the advantages that developers can use. The correct configuration of the application and its environment has been simplified to the maximum, so you don’t need any additional tools to run it. However, if you need to add more functionalities, you can resort to starters – just attach the appropriate library depending on the dependency, and add an annotation. If it were not for the Spring Boot project, this style of microservice architecture wouldn’t have been appreciated so quickly by the IT community from around the world.
If you’d like to develop your skills in Java or IT systems integration, join us!